Python is a versatile, high-level programming language. It is a great first language because the syntax is simple and easy to learn, and it introduces users to general, widely-applicable programming concepts, such as loops and functions. Importantly, it can be applied to address a huge variety of problems, including those related to data science, gaming, automated systems and software. While it is hard to estimate the exact number of users, Python is consistently referenced as one of the top three most globally-used programming languages. The community is huge, so there are plenty of resources and platforms to discuss Python-related issues, and there are a large number of built-in and third-party libraries that make Python applications easy. In the future, we will be discussing various projects related to streaming that implement tools such as the Raspberry Pi. Many of these applications are Python-based or can be used with Python. For example, the Raspberry Pi can be programmed to sync output, such as lighting patterns or personalized audio, to input, such as new Twitch subscribers or donations during a livestream.
The following basics just serve as a reference to Python fundamentals. You’ll need to do your own study and practice on the language, but to make the process easier, we’ve compiled a list of the most essential parts of the language. Knowing these essentials should allow you to write basic code in Python and will provide an overview of the organization of the language. We also address steps that can sometimes sound confusing to individuals with little experience coding, like installing the language, using integrated development environments (IDEs), and downloading different libraries. Aside from learning the language itself, there is a lot of jargon that can seem overwhelming, since specific terms are frequently not explained. It’s not uncommon for skilled programmers to have gaps in their knowledge about some key elements of programming. Oftentimes, searching for information about a specific issue will return suggestions that contain a bunch of new terms, and trying to find exactly what those terms mean leads to a trip down the rabbit hole. Some of this is just part of the learning process, but we hope to provide an overview of commonly overlooked core ideas to help make the journey easier. If there is something that you would like to see covered that is not in this article, feel free to make suggestions in the comments.
Resources
In order to gain an in-depth understanding of the language, experience and hands-on learning are key. After learning the basic logic of the language, some problem solving skills are best gained from troubleshooting. There are many free or cheap interactive courses that teach Python fundamentals alongside hands-on coding, via practice problems or projects. MIT’s Introduction to Computer Science and Programming in Python is a thorough, intensive and completely free option for beginners with no prior coding experience. EdX has sessions of this course, where students can learn along with an instructor. They also have a follow-up course, Introduction to Computational Thinking and Data Science, that teaches problem solving applications related to data science. After learning the logic, syntax, and writing, these fundamental skills can be applied on a higher level, which can involve learning new aspects of the language based on a specific problem. This might include projects like making a calculator or to-do app, analyzing a set of open-source data, or even implementing an algorithm to solve a specific problem, such as finding the shortest travel distance between two points on a map or creating a recommendation system. Google has a variety of free, more specific courses that assume some previous coding knowledge, such as their Machine Learning and Elements of AI courses.
Installing Python
There are multiple ways to install the Python language onto your computer, the most common being via package managers or official Python distributions. The options will slightly vary depending on your computer, experience, and programming goals. Users who want more control and reliability can download the official installer from Python.org.
Official distributions outside of the Python.org download, like Homebrew for MacOS or Microsoft Store applications for Windows, can make the process more user-friendly and manageable. However, there can be a tradeoff depending on what you plan to use them for. For example, the Microsoft Store application’s interface is designed for interactive, simple use, but does not support developers who want to use the application for enterprise-related concerns. Homebrew isn’t directly tied to official Python developers, so updates and changes might not occur alongside the official installer. It also doesn’t support certain packages like Tkinter, which is important for GUI developers.
Anaconda is a different type of free distribution for Python and R. It is a platform that downloads as an application and comes with standard packages and software used with Python. It’s one of the easiest ways to install the language. More specifically, many of the packages are used in scientific computing, such as Pandas and Matplotlib, making it a great choice for data science or just generally working with data that will need to be formatted or analyzed in some way. It comes with a variety of software options, such as several IDE choices (Spyder, Jupyter Notebook, etc.) Anaconda can be downloaded from their website.
Libraries and packages
Basic Python libraries contain pre-written functions that allow a vast number of operations to be conducted, without the user writing the code from scratch. Python comes with a standard library that constructs the language, including general rules like syntax. Python is written in C. However, numerous add-on libraries have been written to increase the functionality of specific tasks. For example, two essential data science libraries include Matplotlib and Pandas. Matplotlib is a library for data plotting and analysis that allow users to conduct higher-level data analysis. It contains functionalities that significantly simplify the process of using tools for data visualization, such as graphs and scatterplots. It is also easy to apply statistical formulations, such as Pearson correlations or euclidean distance measures, as the user does not have to write the function manually and only has to configure the data into a form that the function can process. Pandas streamlines more advanced data formatting, such as reshaping, realignment, grouping and merging of datasets, as well as data filtration and time series functionalities.
Learn the syntax
Knowing the syntax allows you to run code and ensures that the code is written in the correct way, so that it will be read as you intend. This is similar to learning grammar rules in English. In order to write basic code, you should be familiar with the rules pertaining to line and multiline structure, comments, docstrings, indentations, quotations, and keywords versus identifiers.
Printing
The print() function in Python returns a specified message as an output. A classic example for first-time coders is learning how to print “Hello, world!” We can simply place the message inside of the parenthesis, surrounded by quotation marks, and run the code.
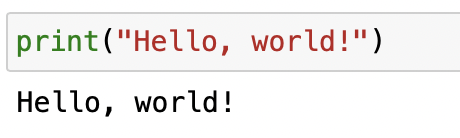
Strings
A string is a data type used to represent text. The character content is surrounded with quotation marks, either single or double. A string can be used literally, meaning directly within the print function, and within variables. Triple quotation marks can be used to make multi-line strings. Because strings are arrays, each value within the string is a single character. We have written the string “Hello, world!” under variable y in our previous examples.
Variables
A variable is a location that stores a data value, created when a value is set equal to a name. This value can then be “called” throughout the program. We have written variables x, y, and z in the following example. X is a numerical variable, and y and z are strings. Multiple objects can be assigned to multiple variables at the same time, and a single object can be assigned to multiple variables. We will discuss each type of variable in more detail, including how each type can be used.
Numbers
Numeric variables do not need to be surrounded in quotation marks like strings. There are three main types of numeric variables in Python; integers, floats, and complex numbers. Integers are whole numbers without a decimal point, floats are numbers with decimal points, and complex numbers are imaginary numbers alongside real numbers.
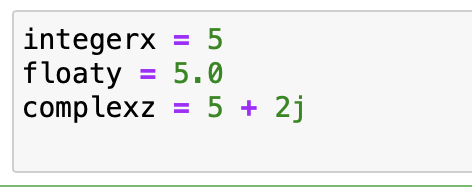
integerx is an integer, floaty is a float, and complexz is a complex number.
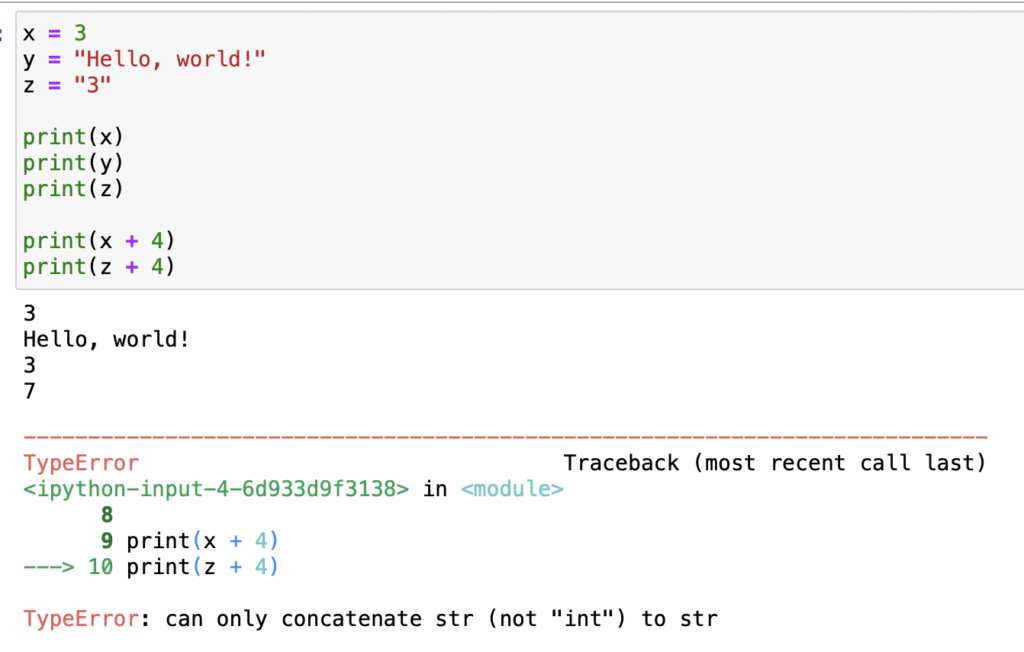
Operations can be performed using the values stored in variables. However, we can see that we get an error in the above example. We are trying to print 5 values, but only receive 4. Our last print statement, z +4, does not work. This is because z is written as a string, and is therefore processed as characters and not a number. In order to perform arithmetic operations on a number, it must be written in one of the three numerical formats. Since the variable x is written as an integer, the operation x + 4 does run and returns 7.
Indentation and comments
Python syntax uses an indentation to separate blocks of code. For example:
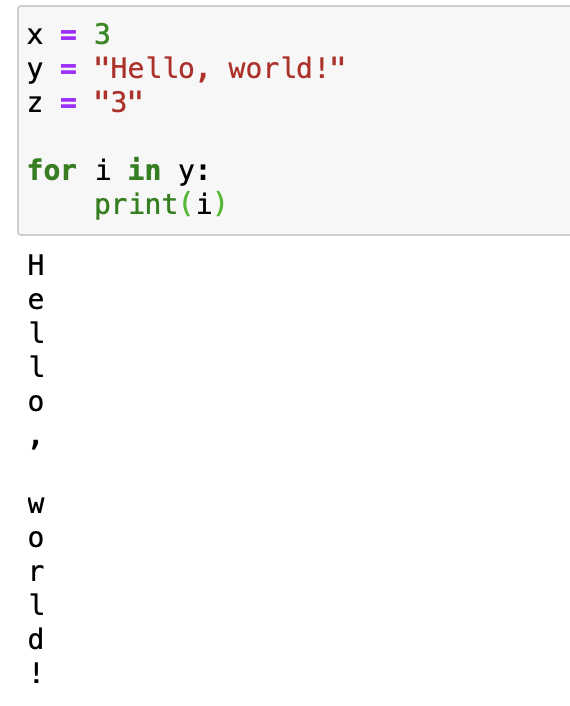
The line containing print(i) is indented. This way, we know that it belongs to the statement above it. The variables x, y and z are each on their own, unindented line. They are global variables that will always be equivalent to their value, unless changed. If we were to move x to be under the for statement, indented the same amount as print(i), x would reset to the value three each time the for loop ran. It would be a local variable. You can also note that printing each item i in a string returns its individual characters, showing that strings are made up of itemized characters.
Comments can be used to add notes to the code, without the notes being confused with the code itself. This can also be helpful for saving pieces of code that need to be debugged or are not ready to run. When a hashtag is used, none of the following text will be run. For example:
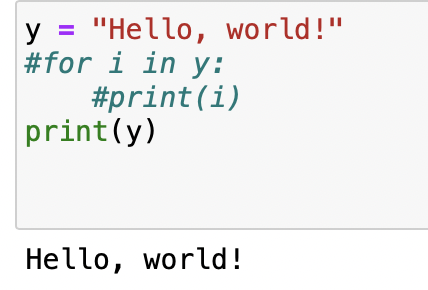
Adding a hashtag to the for statement keeps it from running, and the output will not be printed as it was in the previous example.
Lists
Lists are used to store multiple objects within a single variable, using commas to separate each item and brackets to contain the entirety of the list. The list maintains the order that it is created in, and can be indexed using numbers. A list can contain any type of data, and can store multiple types of data within the same list. Items can be called based on their position. The first item in a list is [0], so calling print(listfruits[0]) in the list of fruits below would return “apple,” and [1] in the list of cars would return 1975. Notice that 1975 and 2007 are numerical values, so they do not need to be put in string format.
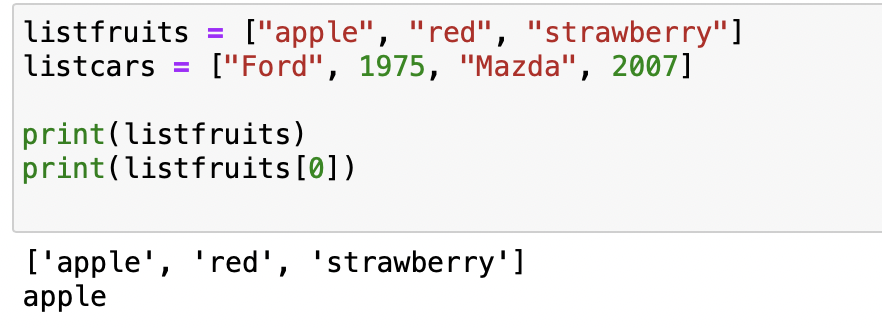
We can add values to a list that has already been created by using append().
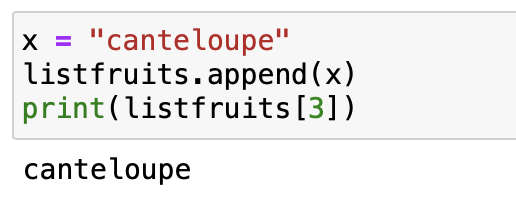
Tuples and sets
Tuples are similar to lists, but are ordered and unchangeable. Parentheses are used to contain the entirety of the tuple. Sets are unordered, unchangeable, cannot contain duplicates, and cannot be indexed. They are written with curly brackets.
Tuples return the same order of values that they were written in. Notice that they also allow “Mazda” to be a value twice, reflecting their allowance of duplicate values.
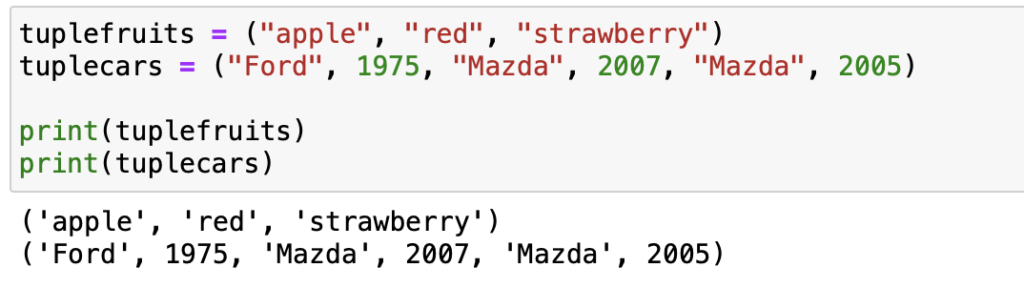
Notice that tuples cannot be changed, and thus, values cannot be added using “append” as they can for lists.
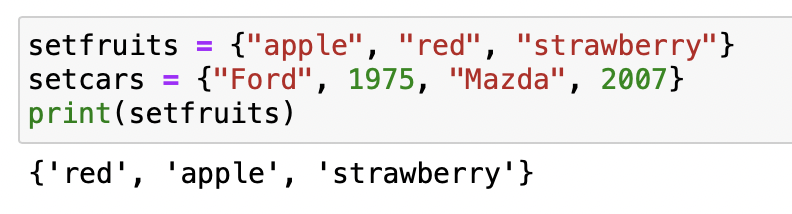
You can see above that sets will return a different order of values than what you may have originally placed in the set. This is because they do not maintain order. A randomly generated order of values in the set will occur each time it is called.
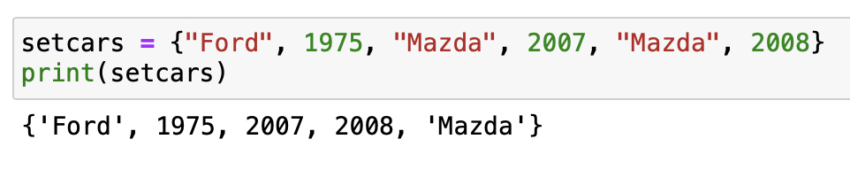
You can also notice that the set will not return the second Mazda referenced in the input. This is reflective of a set’s inability to maintain duplicate values.
Dictionaries
Dictionaries are collections used to store data in pairs of keys and values. A single dictionary can contain multiple keys, and a single key can contain multiple values. Curly brackets specify the start and end of the dictionary, commas separate key/value pairs, and colons separate keys and their corresponding values. A dictionary can contain any type of data, including strings, integers, and lists. Dictionaries are ordered (as of Python 3.7) and cannot contain duplicates. Specific items in the dictionary are called using the name of the key.
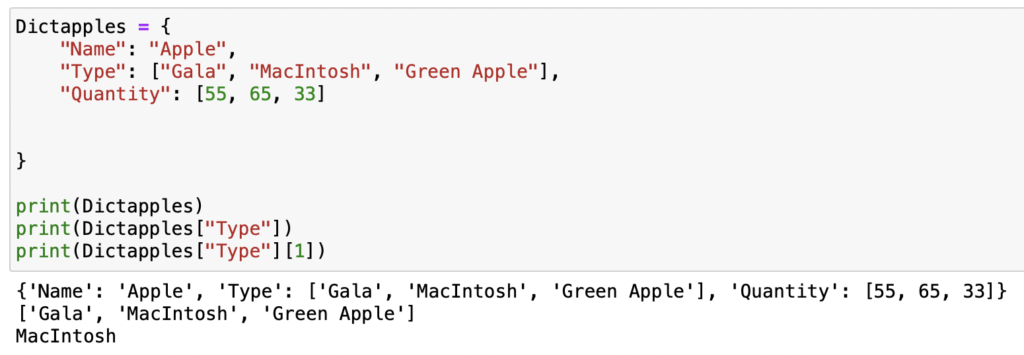
Conditions
Conditional statements allow the use of logical mathematics, such as equal to, less than, and greater than. They can be used in if statements, which allow specific conditions to be represented based on a given situation. For example:
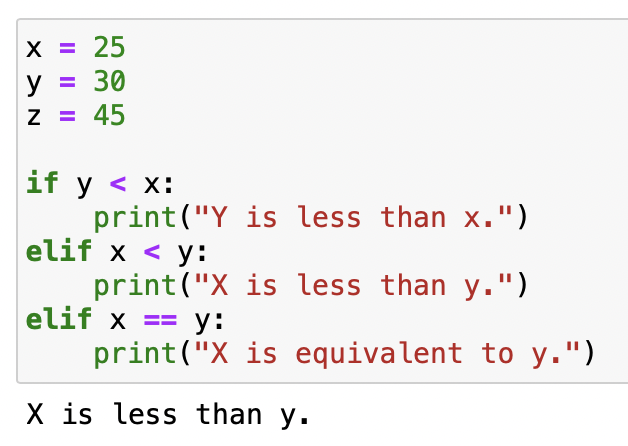
Elif stands for “else if.” If the original statement is not true, it will continue to the elif statement. Because 25 is less than 30, the second print statement is returned. Note that the structure of a conditional statement requires a colon followed by an indentation for all of the code belonging to that statement.
For statements, loops, and for loops
For loops allow a block of code to be run multiple times, based on specific conditions. For example:
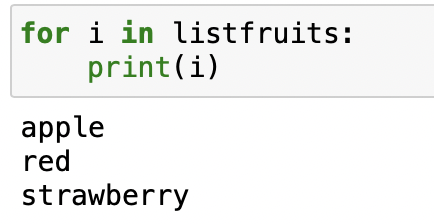
There is an iteration for each item in the list, which is then printed.
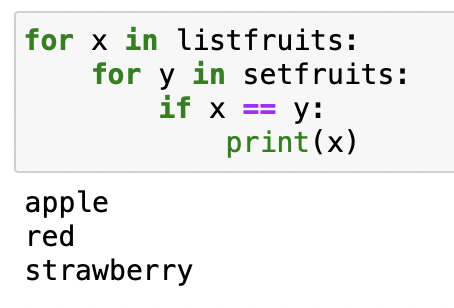
The loop runs a specific amount of times through each item in the list, dictionary, or any iterable object. In our earlier example where we were exploring indentation rules, we saw a basic for loop that iterated through every item in “Hello, World!” In this example, we have a for loop inside of a for loop. Note the additional indentations. This is called a nested for loop. It iterates both through listfruits and setfruits. The outer loop, listfruits, executes 3 times because it contains three items. It executes the inner loop, set fruits, each time. Setfruits also contains three items, and 3 * 3 = 9, so the loop will execute a total of 9 times. After running through all of the items, we can see a list of the items that are contained in both listfruits and setfruits.
Functions
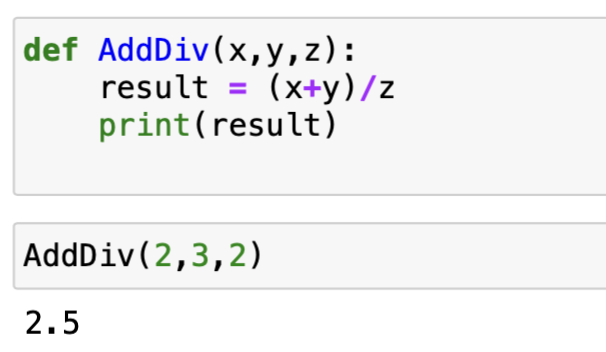
The above is an example of a very simple function. Notice that calling the AddDiv function with three numbers returns the sum of the first two numbers divided by the third number, as specified in the function. We do not have to write the code for addition followed by division manually; instead, we can use this function.
This is a much simpler example what we discussed earlier about the benefits of certain Python libraries, as they contain built-in functions for more complex operations. Rules for functions can be written in an indented code block under def FunctionName():. The variables that will be used to execute the function can be specified within the parenthesis. Because we want to add two numbers and divide by a third here, regardless of what numbers they are, we write the function for this operation with x, y, and z. When we called the function using FunctionName(number x, number y, number z) the values for each of the numbers take the same place as x, y and z do in the original formula.